How to create a canvas drawing tool with vanilla JavaScript
By the end of this tutorial, you will be able to draw different shapes of different colors. Take a look at the final working demo below. Feel free to fork and play around with it! HTML structure The HTML Structure will consist of the following components: A element that will have four drop-down options, namely; freehand, circle, rectangle and eraser An which will define a color picker. A element which will be draw with JavaScript. Here is the HTML structure (as ever, to simplify the process) using Bootstrap. 1 2 3 Drawing Tool 4 5 6 7 8 9 10 Tool: 11 12 Rectangle 13 Freehand 14 Circle 15 Eraser 16 17 18 19 Color: 20 21 22 23 24 25 26 27 For the canvas, we are setting a custom width and height with . The custom setting defines the size and will also ensure that the drawing area is appropriately scaled and allows for precise control over the dimensions of the canvas. Styling with CSS Add the following custom styles: 1 @import url("https://fonts.googleapis.com/css2?family=DM+Mono:ital,wght@0,300;0,400;0,500;1,300;1,400;1,500&display=swap"); 2 body { 3 background-color: rgb(247, 248, 249); 4 font-family: "DM Mono", monospace; 5 } 6 canvas { 7 border: 1px solid rgb(33, 32, 32); 8 border-radius: 10px; 9 background-color: #fff; 10 cursor: crosshair; 11 } 12 h1{ 13 font-weight:600; 14 } The custom styles feature a custom Google font, a border, a border radius to the canvas element, and a white background color. JavaScript functionality Start by getting the canvas element 1 const canvas = document.querySelector("canvas"); Next, create a 2D context object which will allow us to draw in the canvas. The 2D context object contains methods for drawing on the canvas. 1 ctx = canvas.getContext("2d", { willReadFrequently: true }); Define some initial variables: 1 let isDrawing = false; 2 let startX = 0; 3 let startY = 0; 4 let initialImageData; isDrawing : this variable will keep track of when the canvas is being drawn on. startX is the initial point on the X axis on the canvas where any drawing will start. startY is the initial point on the y axis on the canvas where any drawing will start. initialImageData is used to keep a copy of how the canvas looked before any drawing begins. It's also useful for preventing trails when new shapes are drawn. Add event listeners to get the selected color and tool: 1 selectTool = document.getElementById("tool"); 2 3 let currentTool = selectTool.value; 4 5 selectedColor = document.getElementById("drawcolor"); 6 let color = selectedColor.value; 7 8 selectedColor.addEventListener("input", () => { 9 color = selectedColor.value; 10 }); 11 12 selectTool.addEventListener("change", () => { 13 currentTool = selectTool.value; 14 }); Next, add an event listener to the canvas on the mousedown event. The mousedown event will invoke the startDrawing() function. 1 canvas.addEventListener("mousedown", startDrawing); Create the called startDrawing() function which will look like this: 1 function startDrawing(e) { 2 ctx.lineWidth = 5; 3 startX = e.offsetX; 4 startY = e.offsetY; 5 isDrawing = true; 6 ctx.beginPath(); 7 8 ctx.fillStyle = color; 9 ctx.strokeStyle = color; 10 initialImageData = ctx.getImageData(0, 0, canvas.width, canvas.height); 11 } In the code above, on the mousedown event , we will use the linewidth() method provided by the 2D context object to set a custom size for the drawing line width in pixels. isDrawing = true; sets the IsDrawing value to true to signify that the drawing has started. startX = e.offsetX; will set the value of startX to the x-coordinate of the mouse pointer. startY = e.offsetY; will set the value of startY to the y-cordinate of the mouse pointer. ctx.beginPath(); beginPath () is a 2D context method which begins a new path. In this case, a new path will be started at the intersection of startX and startY. ctx.fillStyle = color; will set the color used to fill the drawing ctx.strokeStyle = color; sets the selected color as the stroke color. Next, add an event listener to the canvas on the mousemove event. The mousemove event will invoke the Drawing() function. 1 canvas.addEventListener("mousemove", drawing)
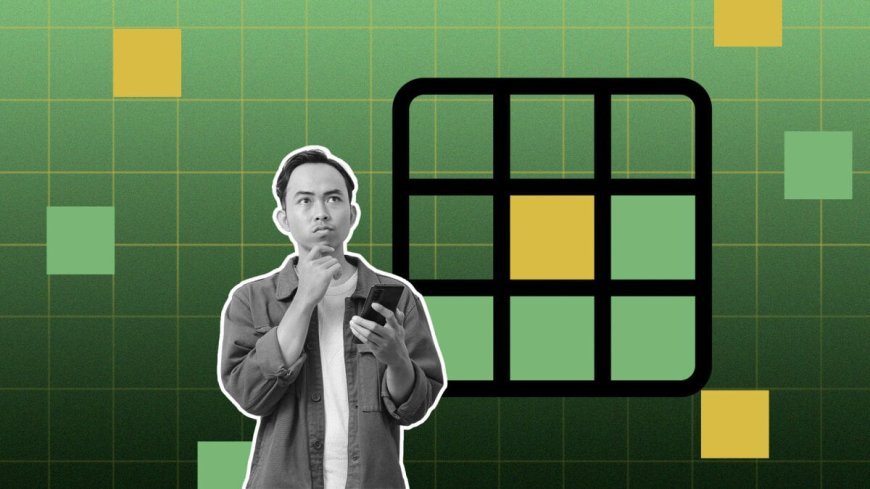
Introduction
In recent years, canvas drawing tools have gained immense popularity among developers and artists alike. These tools allow users to create digital art, sketches, and more, all within their web browsers. This tutorial will guide you through the process of creating a simple canvas drawing tool using vanilla JavaScript. By the end of this article, you'll have a functional drawing application that you can enhance further.
1. Understanding the HTML5 Canvas Element
The HTML5 canvas element is a powerful feature that allows for dynamic, scriptable rendering of 2D shapes and bitmap images. It’s widely used for drawing graphics on the fly and can serve various applications, from games to art projects.
Basic Structure
To start using the canvas, you need to include it in your HTML. Here’s how to set up a simple canvas element:
canvas>
2. Setting Up Your HTML and CSS
Next, let’s set up the HTML and CSS for our drawing tool. You’ll want to give your canvas a little styling to make it visually appealing.
Sample HTML Code
Here’s a basic structure to include a color picker and brush size selector:
Basic CSS Styles
Here’s a simple CSS setup to center your canvas on the page:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
3. Initializing the Canvas in JavaScript
Now, let’s initialize the canvas and set up the drawing context. This is where we’ll configure our drawing environment.
Accessing the Canvas
In your script.js
file, start by accessing the canvas element and setting up the 2D drawing context:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
Basic Functions
You can set up functions to clear and reset the canvas as needed:
function clearCanvas() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
}
4. Implementing Drawing Features
With the canvas set up, let’s implement the core drawing features. We’ll handle mouse events to allow users to draw on the canvas.
Mouse Event Handling
Here’s how you can handle mouse events for drawing:
let drawing = false;
canvas.addEventListener('mousedown', (e) => {
drawing = true;
ctx.beginPath();
ctx.moveTo(e.offsetX, e.offsetY);
});
canvas.addEventListener('mousemove', (e) => {
if (drawing) {
ctx.lineTo(e.offsetX, e.offsetY);
ctx.stroke();
}
});
canvas.addEventListener('mouseup', () => {
drawing = false;
});
Brush Feature
Add the ability to change brush colors and sizes with the following code:
const colorPicker = document.getElementById('colorPicker');
const brushSize = document.getElementById('brushSize');
ctx.strokeStyle = colorPicker.value;
ctx.lineWidth = brushSize.value;
colorPicker.addEventListener('input', () => {
ctx.strokeStyle = colorPicker.value;
});
brushSize.addEventListener('input', () => {
ctx.lineWidth = brushSize.value;
});
5. Enhancing Your Drawing Tool
To make your drawing tool more robust, consider adding features like undo/redo functionality and saving drawings.
Advanced Features
You can implement a simple undo feature by storing the canvas state in an array and reverting to the last state when requested.
Color Picker and Brush Size Slider
Integrating a color picker and a brush size slider improves user interaction. The examples given earlier showcase how to implement these features.
6. Final Touches and Improvements
Enhancing user experience can make your tool more engaging. Consider adding buttons for features like "Clear Canvas," "Undo," and "Save."
Suggested Features
- Clear Button: A button to reset the canvas.
- Save Button: Allows users to download their artwork.
Example code for a clear button:
<button id="clearButton">Clear Canvasbutton>
And in your JavaScript:
document.getElementById('clearButton').addEventListener('click', clearCanvas);
Conclusion
Congratulations! You’ve built a simple yet functional canvas drawing tool using vanilla JavaScript. This foundational project can serve as a stepping stone for more advanced features and enhancements. As you continue to explore, consider experimenting with more sophisticated functionalities to make your drawing application even more engaging.
FAQ Section
1. What is the HTML5 canvas?
The HTML5 canvas is an element that allows for dynamic rendering of graphics and images on the web.
2. How do I start drawing on the canvas?
You can start drawing by implementing mouse event listeners to track mouse movements and draw on the canvas.
3. Can I save my drawings?
Yes, you can save your drawings by converting the canvas content to an image using canvas.toDataURL()
.
4. What JavaScript functions are used for canvas drawing?
Common functions include beginPath()
, moveTo()
, lineTo()
, and stroke()
.
5. How can I change the brush size?
You can change the brush size by adding a range input and updating the lineWidth
property of the canvas context.
6. Is it possible to add more colors?
Absolutely! You can use an to let users select different colors for their brushes.
7. How do I improve my drawing tool further?
You can add features like shapes, layers, and export options to enhance your drawing tool.
What's Your Reaction?



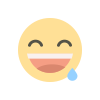

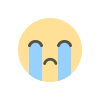
